Creating an Interactive 👁 Eye Animation with JavaScript

In the world of web development, adding interactive elements to your website can greatly enhance user experience. One fun and engaging effect you can create is a moving eye that follows the user’s mouse cursor. In this article, we’ll explore how to achieve this effect using HTML, CSS, and JavaScript.
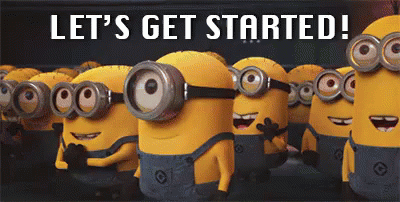
Here’s a step-by-step guide on how to do it:
Step 1: Create the HTML Structure
First, let’s set up the basic HTML structure for our eye animation, create a eye
container with a div eye-ball
<div id="eye">
<div id="eyeBall"></div>
</div>
Step2: CSS Styling
Next, let’s add some CSS to style our eye animation. We’ll use a simple design with a white eye and a black eyeball to create a high-contrast effect
@import url('https://fonts.googleapis.com/css2?family=Mate+SC&display=swap');
body {
background: #63636e;
text-align: center;
}
h1 {
font-family: Mate Sc;
font-size: 3em;
color: white;
}
#eye {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
height: 250px;
width: 250px;
background: white;
border-radius: 50%;
overflow: hidden;
box-shadow: 0 0 30px 1px #222236ad;
}
#eyeBall {
position: absolute;
top: 50%;
left: 50%;
height: 70px;
width: 70px;
transform: translate(-50%, -50%);
background: black;
border-radius: 50%;
transition: 0;
}
Step3: JavaScript Interaction
Now we will add life to our project. We are going to use onmousemove
and onmouseout
events.
Tracking Mouse Movement with
onmousemove
document.onmousemove = (event) => {
// Get the mouse cursor's coordinates
var x = event.clientX * 100 / window.innerWidth + "%";
var y = event.clientY * 100 / window.innerHeight + "%";
// Update the element's position based on the mouse coordinates
eyeBall.style.transition = "0s";
eyeBall.style.left = x;
eyeBall.style.top = y;
}
The onmousemove
event is triggered whenever the mouse cursor moves over the document. In the example above, we're using this event to update the position of an element (eyeBall
) based on the mouse's coordinates. This creates a cool effect where the element follows the mouse cursor as you move it around the page.
Restoring Element Position with
onmouseout
document.onmouseout = (event) => {
// Reset the element's position to the center of the window
eyeBall.style.transition = "0.7s";
eyeBall.style.left = "50%";
eyeBall.style.top = "50%";
}
The onmouseout
event is triggered when the mouse cursor leaves the document. In our example, we're using this event to smoothly transition the element (eyeBall
) back to the center of the window when the mouse cursor is no longer hovering over it.
By combining these two events, you can create dynamic and interactive elements on your webpage that respond to the user’s mouse movements. Experiment with different elements and effects to add a touch of magic to your website! ✨
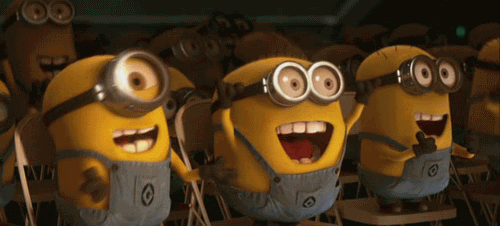
Thank you for reading this article. If you found this helpful and interesting, please clap and follow me for more such content.
you can also check out my new app on playstore here
must Have chrome extension if you are applying for job click here
If I got something wrong, mention it in the comments. I would love to improve.